Updating Custom Metadata/Descriptions for BI Objects
Overview
In this guide, we will walk you through on how to update the descriptions on BI Folders. These steps will work for any BI object (Report, Data Source, BI Server, and etc.). Let's assume we want to update the description of a folder that's on our Tableau BI server. This guide will work for any BI source that's been cataloged by Alation.
APIs being used
- BI Source (GBMv2) Overview
- Custom Field
- Custom Field Values Overview
- APIAccessToken <- This guide assumes you have a valid access token
Permissions Needed
You will need a Server Admin role to do these steps. You can check the APIs by Roles for more details.
Completed Code
Below is the completed python code for this guide.
1. Identify which object(s) we want to update
Get BI Server ID
We can use GET a list of BI Servers to identify the BI Server ID that Alation uses. This can also be found in the URL.
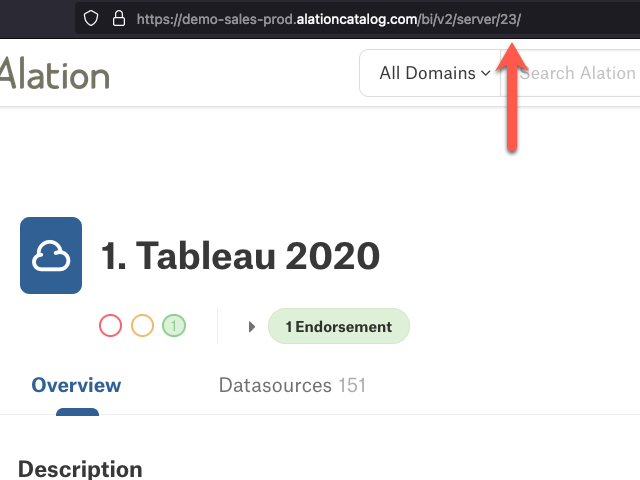
# if you get an error, on the command line type pip install requests
import requests
# setting the base_url so that all we need to do is swap API endpoints
base_url = "CHANGEME"
# api access key
api_key = "CHANGEME"
# setting up this access key to be in the headers
headers = {"token": api_key}
# api for bi server endpoint
bi_server_api = "/integration/v2/bi/server/"
bi_server_uri = "https://tableau2020."
# make the API call
res = requests.get(base_url + bi_server_api, headers=headers)
# convert the response to a python dict.
bi_servers = res.json()
# iterate through our servers to find the one we need
bi_server_id = 0
# would recommend creating a function for this action
# vs what we're doing in this guide
for serv in bi_servers:
# matching on URI to find the one that starts with what we need
if serv["uri"].startswith(bi_server_uri):
bi_server_id = serv["id"] # in this example, our bi server ID = 23
print("The BI folder id for \"{}\" is {}". format(serv['title'], bi_server_id))
break
Get the BI Folders
We can use the GET a list of folders from a specified BI Server on this BI Server. Now a folder in Alation can represent projects in Tableau, Work Spaces in PowerBI, and etc. Browse your BI Server and Alation to get a feel for how it is organized in Alation.
# this code is a continuation of the above code.
bi_folder_name = "Ticket Sales"
bi_folder_api = "/integration/v2/bi/server/{}/folder/".format(bi_server_id)
res = requests.get(base_url + bi_folder_api, headers=headers)
bi_folders = res.json()
bi_folder_id = 0
for folder in bi_folders:
# matching on folder name to find the one that starts with what we need
if folder["name"].startswith(bi_folder_name):
bi_folder_id = folder["id"] # in this example, our bi folder ID = 744
print("The BI folder id for \"{}\" is {}". format(bi_folder_name, bi_folder_id))
break
2. Get the Custom Field IDs
We need to get the custom field ID for our "Description", singular name, custom field. The "Description" field is also a "Rich Text" type. If you wanted to update more custom fields on a BI source, then get those additional IDs here. GET multiple Custom Fields
In our URL parameters, we will specify the field type as "RICH_TEXT".
needed_cf_type = "RICH_TEXT"
singular_field_name = "Description" # the custom field name we're looking for
custom_field_api = "/integration/v2/custom_field/?field_type={}&limit=100&skip=0".format(needed_cf_type)
res = requests.get(base_url + custom_field_api, headers=headers)
custom_fields = res.json()
custom_field_id = 0
for cf in custom_fields:
# matching on folder name to find the one that starts with what we need
if cf["name_singular"] == (singular_field_name):
custom_field_id = folder["id"] # in this example, our bi folder ID = 4
print("The custom field \"{}\" the ID is {}". format(singular_field_name, custom_field_id))
break
3. Update the description
Alright! We have all of the needed ids. Let's go to update the Description.
NOTE: When updating Rich Text Fields, make sure you review HTML Sanitization for which HTML values are allowed. iframes are always a good work around when needed ;)
Before API Update
Here's our "Folder" right before the API call.
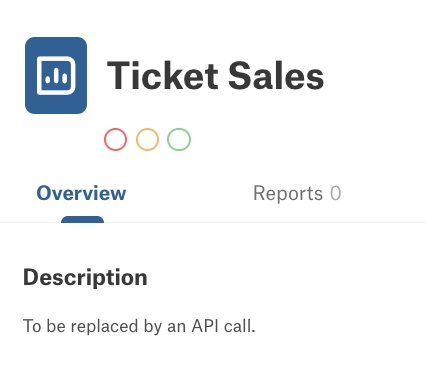
Update Call
custom_field_value_api = "/integration/v2/custom_field_value/"
data = [
{
"field_id": custom_field_id,
"otype": "bi_folder",
"oid": bi_folder_id,
"value": "<ul><li>Lorem ipsum dolor sit amet, consectetuer adipiscing elit.</li><li>Aliquam tincidunt mauris eu risus.</li><li>Vestibulum auctor dapibus neque.</li></ul>"
}
]
# Requests library makes it easy for us to convert a payload to json.
res = requests.put(base_url + custom_field_value_api, headers=headers, json=data)
print(res.json())
# if all went according to plan then, you should see a response that looks like
# {'updated_field_values': 1, 'new_field_values': 0, 'field_values_received': 1}
After API Call
And now we can see the updated description!
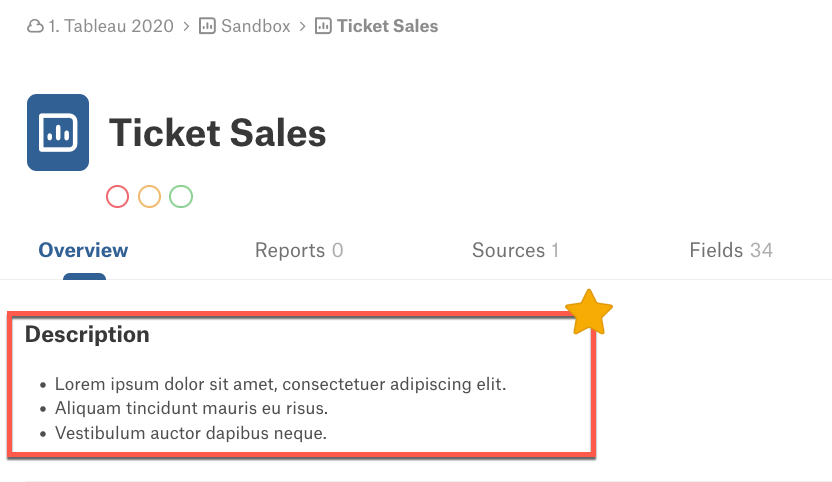
And as always....
Updated 9 months ago